I think React Hooks was a bit confusing in the beginning. It took me some time and a lot of articles to get the drift.
This article contains what I would wish to find when first started reading about hooks.
So this is it: Key points of React Hooks explained as clear and simple as I could make it.
What are hooks and what’s changing?
"Hooks are functions that let you 'hook into' React state and lifecycle features from function components."
— React
Below are what I consider the biggest changes:
- Classes: Hooks have the ambition to replace all use cases of classes
- State: State is being “hooked” by the hook;useState, instead of being set by a constructor
- Redux: Global state will solve some of the use cases Redux is used for. Redux adapts to the changes as well. The current Redux store state is propagated through React’s new createContext API. Note that there will be improvements.
- This: “This” disappears
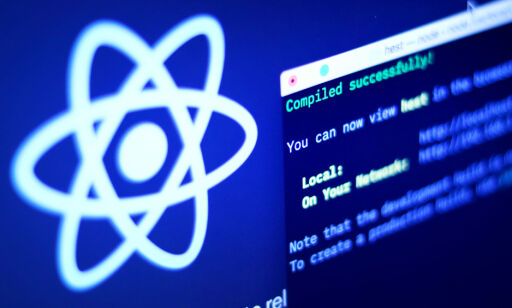
Dette er nytt i Create React App 2
- Sårt etterspurte features for mange.
And there are no breaking changes. Your application would work just fine as it is with the new update.
Okey, so we know the “purpose” of this change, but how do we use hooks?
I work on a medium sized project with states, props and classes all over. How can I easily update the project? Preferably component by component, and not being forced to destroy the whole project and build it one more time.
Where did props go?
I think there is a small gap in the documentation from React when it comes to props. They had this simple component which had a count and the state was set within the component by using useState. What I needed to know was how to set props passed from the parent.
This was easy. It’s just the same, but without “this”. Probably why they didn’t bother to mention it.
// Parent:
export default () => {
return (
<Component myProp={'hello world'} />
);
};
// Child:
const component = (props) => {
return (
<div>{props.myProp}</div>
);
};
export const component;
State
Over to state. This was well documentation on React’s pages, so I’m just gonna grab their code example:
import React, { useState } from 'react';
function Example() {
// Declare a new state variable, which we'll call "count"
const [count, setCount] = useState(0);
The state is being set by the hook useState. You can declare the state without wrapping it in an array as well.
Update state
You update the state by “setState”. It accepts a new state value and enqueues a re-render of the component. Using the example from above it could look like this:
const [count, setCount] = useState(0);
const newState = 9;
setCount(newState);
States from Redux
I’m going to slowly migrate to using hooks component by component, so I just want to fetch data from the Redux store as I did before with connect. Well, you can do exactly that while waiting for something better from Redux. (Link to the road map on React-Redux)
Or you could make a store.js-file, import the redux store and use useContextlike so:
import { Store } from './store.js';
function Example() {
const state = useContext(Store);
return (
<div>
{state.data}
</div>
)
Here’s an example of how you can Rebuild Redux with Hooks and Context.

Dette misliker folk med React
ForrigeUke: Upopulære meninger, React Router 5, tab-komponent, hooks-routing og React.warn/React.error.
Can you make a child component that uses hooks without updating the parent?
Yes, but remember that react-dom and react must be of matching versions and you need to have the same version of React throughout the application (16.8.0 or above). If not you will meet this error:
Uncaught Invariant Violation: Hooks can only be called inside the body of a function component.
(You might want to update react-test-renderer too)
Hope you’re ready to jump into learning more about Hooks and will start using it soon!
Further reading: Introducing Hooks, reactjs.org
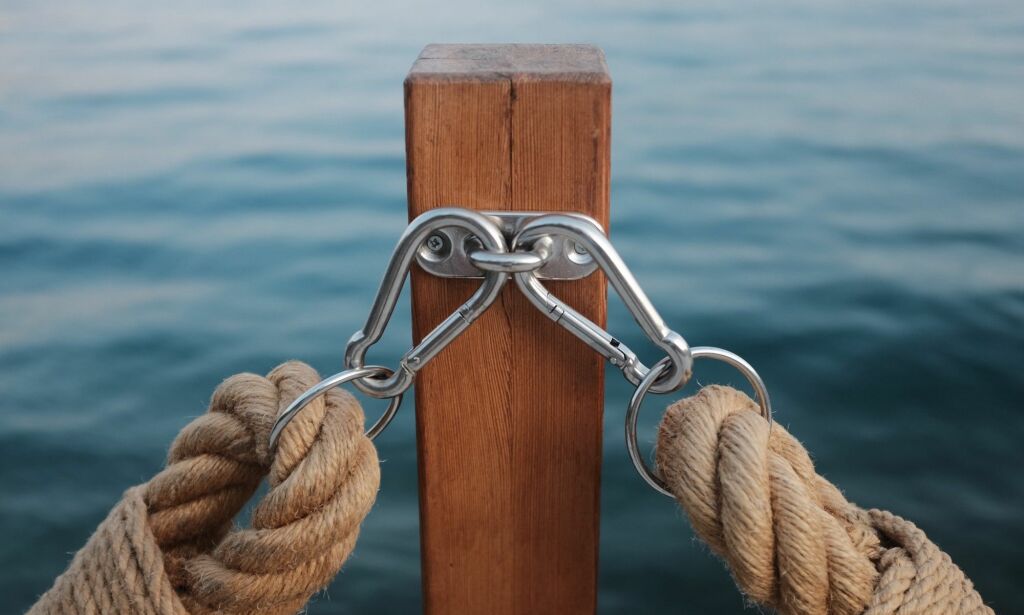
Slik bruker du nye React Hooks
React Hooks er her for å bli — dette er hva det betyr for deg.